我自己曾基于 Spring Framework 的缓存抽象实现了使用 Memcached 分布式缓存方案的缓存工具,并正在目前的项目中使用,至今尚未发现过什么问题,但我清楚该实现还是存在很多不完善之处的。由于在 Redisson 中发现它也实现了使用 Redis 作为分布式缓存方案的这样一套工具,所以打算学习一下它的实现,应该能获益不少。
Spring Framework 的缓存抽象工具
Spring Framework 从 3.1 版本开始提供了缓存抽象工具,该工具可以透明的在使用 Spring 的应用中添加缓存机制,不会使应用的实现对具体的缓存方案产生依赖,因此无需修改应用实现就能够方便的替换缓存方案。
Spring 提供的缓存抽象主要基于 org.springframework.cache.Cache
和 org.springframework.cache.CacheManager
两个接口。开发者可以实现这两个接口来支持各种不同的缓存方案。而在应用中只要使用如下注解即可声明式的使用缓存了,
-
@Cacheable
触发填写缓存,例如:将方法返回值写入缓存系统 -
@CacheEvict
触发移除缓存 -
@CachePut
在不妨碍方法执行的前提下更新缓存 -
@Caching
在一个方法上将多个缓存操作组合执行 -
@CacheConfig
在类级别上添加共享的缓存配置
Spring Framework 中也提供了几个已经实现好的缓存方案,分别是基于:
- java.util.concurrent.ConcurrentMap
- EhCache
- Gemfire cache
- Caffeine
- Guava caches
- JSR-107 compliant caches
如果其中有你正好要用的缓存方案,那么就可以直接拿来用了。可以在 sping-context
和 spring-context-support
中的 org.springframework.cache
包中找到它们。
对 Spring 缓存抽象就不多做介绍了,想要详细了解建议直接阅读其参考文档。
Spring 缓存抽象的主要接口
通常开发者只需要实现上面说的 org.springframework.cache.Cache
和 org.springframework.cache.CacheManager
两个接口就可以完成对一个新的缓存方案的支持,下面来看看这两个接口的主要内容。
org.springframework.cache.Cache
该接口中有以下主要方法声明,
/** 获取 cache 名称 */
String getName();
/** 获取真正的缓存提供方案 */
Object getNativeCache();
/** 从缓存中获取 key 对应的值(包含在一个 ValueWrapper 中)
ValueWrapper get(Object key);
/** 从缓存中获取 key 对应的指定类型的值(4.0版本新增) */
<T> T get(Object key, Class<T> type);
/**
* 从缓存中获取 key 对应的值,如果缓存没有命中,则添加缓存,
* 此时可异步地从 valueLoader 中获取对应的值(4.3版本新增)
*/
<T> T get(Object key, Callable<T> valueLoader);
/** 缓存 key-value,如果缓存中已经有对应的 key,则替换其 value */
void put(Object key, Object value);
/** 缓存 key-value,如果缓存中已经有对应的 key,则返回已有的 value,不做替换 */
ValueWrapper putIfAbsent(Object key, Object value);
/** 从缓存中移除对应的 key */
void evict(Object key);
/** 清空缓存 */
void clear();
org.springframework.cache.CacheManager
该接口中有以下主要方法声明,
/** 根据指定的名称获取 cache 实例 */
Cache getCache(String name);
/** 返回当前 cacheManager 已知的所有缓存名称 */
Collection<String> getCacheNames();
使用 Redisson 的 Spring cache 实现
这里假设你已经使用过其他的缓存方案,即应用中已经添加了 Spring cache 的相关注解,此时要使用 Redis 作为新的缓存方案,那么使用 Redisson 只要进行如下配置即可。
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:cache="http://www.springframework.org/schema/cache"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/cache
http://www.springframework.org/schema/cache/spring-cache.xsd">
<cache:annotation-driven />
<bean id="cacheManager"
class="org.redisson.spring.cache.RedissonSpringCacheManager">
<property name="redisson" ref="redissonClient" />
<property name="config">
<map>
<entry key="testCache">
<bean class="org.redisson.spring.cache.CacheConfig">
<property name="TTL" value="1800000" /> <!-- 30 minutes -->
<property name="maxIdleTime" value="900000" /> <!-- 15 minutes -->
</bean>
</entry>
</map>
</property>
</bean>
</beans>
最近越来越不喜欢 Spring 的 XML 配置方式了,不仅阅读起来噪音太多,配置起来也觉得繁琐,所以再给出一份编码方式的配置,配置的内容和上面的 XML 是一样的。
@Configuration
@EnableCaching
public class CacheConfig {
@Autowired
RedissonClient redissonClient;
@Bean
CacheManager cacheManager() {
Map<String, CacheConfig> config = new HashMap<String, CacheConfig>();
config.put("testCache", new CacheConfig(30*60*1000, 15*60*1000));
return new RedissonSpringCacheManager(redissonClient, config);
}
}
在了解其使用方法后,接下来我们就进入正题,看看 Redisson 到底是如何实现 Spring cache 的,由于篇幅较长,这块内容之后另起一篇。
本文同时发布于我的微信订阅号
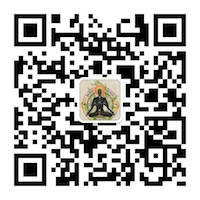